Working with Tables
Extracting Plain Text from the Table of OneNote Document
Aspose.Note for JAVA offers the Document class that represents a OneNote file. The Document class exposes the getChildNodes method that can be called to extract table nodes from a OneNote document.
This article shows how to:
- Get table text from a OneNote document.
- Get row text from a table in a OneNote document.
- Get cell text from a row in a table.
Get Table Text from OneNote Document
This example works as follows:
- Create an object of the Document class.
- Call getChildNodes method of the Document class.
- Retrieve a list of table nodes.
- Call the stream-based code to extract text.
- Display text on the output screen.
The following example shows how to get table text from a OneNote document.
1String dataDir = Utils.getSharedDataDir(ExtractTextFromTable.class) + "tables/";
2
3// Load the document into Aspose.Note
4Document document = new Document(dataDir + "Sample1.one");
5
6// Get a list of table nodes
7List<Table> nodes = document.getChildNodes(Table.class);
8
9for (int i = 0; i < nodes.size(); i++) {
10 Table table = nodes.get(i);
11 System.out.println("Table # " + i);
12
13 // Retrieve text
14 List<RichText> textNodes = (List<RichText>) table.getChildNodes(RichText.class);
15 StringBuilder text = new StringBuilder();
16 for (RichText richText : textNodes) {
17 text = text.append(richText.getText().toString());
18 }
19
20 // Print text on the output screen
21 System.out.println(text);
22}
Get Row Text from a Table in a OneNote Document
This example works as follows:
- Create an object of the Document class.
- Filter out a list of table nodes.
- Iterate through table rows.
- Call the stream-based code to extract text.
- Display the text on the output screen.
The following example shows how to extract row text from a table in a OneNote document.
1String dataDir = Utils.getSharedDataDir(ExtractRowtextfromtableinOneNotedocument.class) + "tables/";
2
3// Load the document into Aspose.Note.
4Document document = new Document(dataDir + "Sample1.one", new LoadOptions());
5
6// Get a list of table nodes
7List<Table> nodes = (List<Table>) document.getChildNodes(Table.class);
8
9// Set row count
10int rowCount = 0;
11
12for (Table table : nodes) {
13 // Iterate through table rows
14 for (TableRow row : table) {
15 rowCount++;
16 // Retrieve text
17 List<RichText> textNodes = (List<RichText>) row.getChildNodes(RichText.class);
18 StringBuilder text = new StringBuilder();
19 for (RichText richText : textNodes) {
20 text = text.append(richText.getText().toString());
21 }
22
23 // Print text on the output screen
24 System.out.println(text);
25 }
26}
Get Cell Text from a Row in a Table
This example works as follows:
- Create an object of the Document class.
- Filter out a list of table nodes.
- Iterate through the table rows.
- Filter out a list of cell nodes from each row.
- Iterate through the row cells.
- Call the stream-based code to extract text.
- Display the text on the output screen.
The following example shows how to get cell text from a row of the table.
1String dataDir = Utils.getSharedDataDir(GetCellTextFromRowOfTable.class) + "tables/";
2
3// Load the document into Aspose.Note.
4Document document = new Document(dataDir + "Sample1.one");
5
6// Get a list of table nodes
7List<Table> nodes = (List<Table>) document.getChildNodes(Table.class);
8
9
10for (Table table : nodes) {
11 // Iterate through table rows
12 for (TableRow row : table) {
13 // Get list of TableCell nodes
14 List<TableCell> cellNodes = (List<TableCell>) row.getChildNodes(TableCell.class);
15 // iterate through table cells
16 for (TableCell cell : cellNodes) {
17 // Retrieve text
18 List<RichText> textNodes = (List<RichText>) cell.getChildNodes(RichText.class);
19 StringBuilder text = new StringBuilder();
20 for (RichText richText : textNodes) {
21 text = text.append(richText.getText().toString());
22 }
23
24 // Print text on the output screen
25 System.out.println(text);
26 }
27 }
28}
Insert a Table in OneNote Document
Aspose.Note for Java APIs allows developers to insert a table at a particular node position. This article is meant to show you how to create a table in OneNote document programmatically.
Aspose.Note for Java offers the Document class that represents a OneNote file. Developers can append content under the TableCell node, table cells to the TableRow node, table row to the Table node. Later they could append table under OutlineElement node, outline element to Outline node, outline to Page node and then a page to the Document node. It’s all based on the Aspose.Note DOM structure.
The following example shows how to insert a table in a OneNote document.
1// The path to th documents directory.
2String dataDir = Utils.getSharedDataDir(InsertTable.class) + "tables\\";
3
4Document doc = new Document();
5
6// Initialize Page class object
7Page page = new Page(doc);
8
9// Initialize TableRow class object
10TableRow row1 = new TableRow(doc);
11
12// Initialize TableCell class objects
13TableCell cell11 = new TableCell(doc);
14TableCell cell12 = new TableCell(doc);
15TableCell cell13 = new TableCell(doc);
16
17// Append outline elements in the table cell
18cell11.appendChildLast(GetOutlineElementWithText(doc, "cell_1.1"));
19cell12.appendChildLast(GetOutlineElementWithText(doc, "cell_1.2"));
20cell13.appendChildLast(GetOutlineElementWithText(doc, "cell_1.3"));
21
22// Table cells to rows
23row1.appendChildLast(cell11);
24row1.appendChildLast(cell12);
25row1.appendChildLast(cell13);
26
27// Initialize TableRow class object
28TableRow row2 = new TableRow(doc);
29
30// initialize TableCell class objects
31TableCell cell21 = new TableCell(doc);
32TableCell cell22 = new TableCell(doc);
33TableCell cell23 = new TableCell(doc);
34
35// Append outline elements in the table cell
36cell21.appendChildLast(GetOutlineElementWithText(doc, "cell_2.1"));
37cell22.appendChildLast(GetOutlineElementWithText(doc, "cell_2.2"));
38cell23.appendChildLast(GetOutlineElementWithText(doc, "cell_2.3"));
39
40// Append table cells to rows
41row2.appendChildLast(cell21);
42row2.appendChildLast(cell22);
43row2.appendChildLast(cell23);
44
45// Initialize Table class object and set column widths
46Table table = new Table(doc);
47table.setBordersVisible(true);
48TableColumn col = new TableColumn();
49col.setWidth(200);
50
51table.getColumns().addItem(col);
52table.getColumns().addItem(col);
53table.getColumns().addItem(col);
54
55// Append table rows to table
56table.appendChildLast(row1);
57table.appendChildLast(row2);
58
59// Initialize Outline object
60Outline outline = new Outline(doc);
61
62// Initialize OutlineElement object
63OutlineElement outlineElem = new OutlineElement(doc);
64
65// Add table to outline element node
66outlineElem.appendChildLast(table);
67
68// Add outline element to outline
69outline.appendChildLast(outlineElem);
70
71// Add outline to page node
72page.appendChildLast(outline);
73
74// Add page to document node
75doc.appendChildLast(page);
76dataDir = dataDir + "InsertTable_out.one";
77doc.save(dataDir);
Create a Table with Locked Columns in the OneNote Document
Aspose.Note for Java APIs allows developers to insert a table at a particular node position. This article is meant to show you how to create a table with a locked column in OneNote document programmatically.
Aspose.Note for Java offers the Document class that represents a OneNote file. Developers can append content under the TableCell node, table cells to the TableRow node, table row to the Table node. LockedWidth property of the Table class allows bolting its width. Later they could append table under OutlineElement node, outline element to Outline node, outline to Page node and then a page to the Document node. It’s all based on the Aspose.Note DOM structure.
The following example shows how to insert a table with locked columns in a OneNote document.
1 String dataDir = Utils.getSharedDataDir(CreateTableWithLockedColumns.class) + "tables\\";
2
3 // Create an object of the Document class
4 Document doc = new Document();
5
6 // Initialize Page class object
7 Page page = new Page(doc);
8
9 // Initialize TableRow class object
10 TableRow row1 = new TableRow(doc);
11
12 // Initialize TableCell class object and set text content
13 TableCell cell11 = new TableCell(doc);
14 cell11.appendChildLast(InsertTable.GetOutlineElementWithText(doc, "Small text"));
15 row1.appendChildLast(cell11);
16
17 // Initialize TableRow class object
18 TableRow row2 = new TableRow(doc);
19
20 // Initialize TableCell class object and set text content
21 TableCell cell21 = new TableCell(doc);
22 cell21.appendChildLast(InsertTable.GetOutlineElementWithText(doc, "Long text with several words and spaces."));
23 row2.appendChildLast(cell21);
24
25 // Initialize Table class object
26 Table table = new Table(doc);
27 table.setBordersVisible(true);
28
29 TableColumn col = new TableColumn();
30 col.setWidth(200);
31 col.setLockedWidth(true);
32
33 table.getColumns().addItem(col);
34
35 // Add rows
36 table.appendChildLast(row1);
37 table.appendChildLast(row2);
38
39 Outline outline = new Outline(doc);
40 OutlineElement outlineElem = new OutlineElement(doc);
41
42 // Add table node
43 outlineElem.appendChildLast(table);
44
45 // Add outline element node
46 outline.appendChildLast(outlineElem);
47
48 // Add outline node
49 page.appendChildLast(outline);
50
51 // Add page node
52 doc.appendChildLast(page);
53 dataDir = dataDir + "CreateTableWithLockedColumns_out.one";
54 doc.save(dataDir);
GetOutlineElementWithText Method
1public static OutlineElement GetOutlineElementWithText(Document doc, String text)
2{
3 OutlineElement outlineElem = new OutlineElement(doc);
4 ParagraphStyle textStyle = new ParagraphStyle();
5 textStyle.setFontColor(Color.BLACK);
6 textStyle.setFontName("Arial");
7 textStyle.setFontSize(10);;
8
9 RichText richText = new RichText(doc);
10 richText.setText(text);
11 richText.setParagraphStyle(textStyle);
12
13 outlineElem.appendChildLast(richText);
14 return outlineElem;
15}
Setting Cell Background Color
1// Load the document into Aspose.Note.
2Document doc = new Document();
3
4// Initialize TableRow class object
5TableRow row1 = new TableRow(doc);
6// Initialize TableCell class object and set text content
7TableCell cell11 = new TableCell(doc);
8cell11.appendChildLast(GetOutlineElementWithText(doc, "Small text"));
9cell11.setBackgroundColor(Color.BLACK);
10row1.appendChildLast(cell11);
Changing style of a table
1 private static void setRowStyle(TableRow row, Color highlightColor, boolean bold, boolean italic) {
2 for (TableCell cell: row)
3 {
4 cell.setBackgroundColor(highlightColor);
5
6 for (RichText node: cell.getChildNodes(RichText.class))
7 {
8 node.getParagraphStyle().setBold(bold);
9 node.getParagraphStyle().setItalic(italic);
10
11 for (TextStyle style: node.getStyles())
12 {
13 style.setBold(bold);
14 style.setItalic(italic);
15 }
16 }
17 }
18 }
19
20 public static void main(String... args) throws IOException {
21 String dataDir = Utils.getSharedDataDir(CreateTableWithLockedColumns.class) + "tables\\";
22
23 // Load the document into Aspose.Note.
24 Document document = new Document(dataDir + "ChangeTableStyleIn.one");
25
26 // Get a list of table nodes
27 List<Table> nodes = document.getChildNodes(Table.class);
28
29 for (Table table: nodes)
30 {
31 setRowStyle(table.getFirstChild(), Color.GRAY, true, true);
32
33 // Highlight first row after head.
34 boolean flag = false;
35 List<TableRow> rows = table.getChildren();
36 for (int i = 1; i < rows.size(); ++i)
37 {
38 setRowStyle(rows.get(i), flag ? Color.lightGray : new java.awt.Color(-1, true), false, false);
39
40 flag = !flag;
41 }
42 }
43
44 document.save(Paths.get(dataDir, "ChangeTableStyleOut.one").toString());
45 }
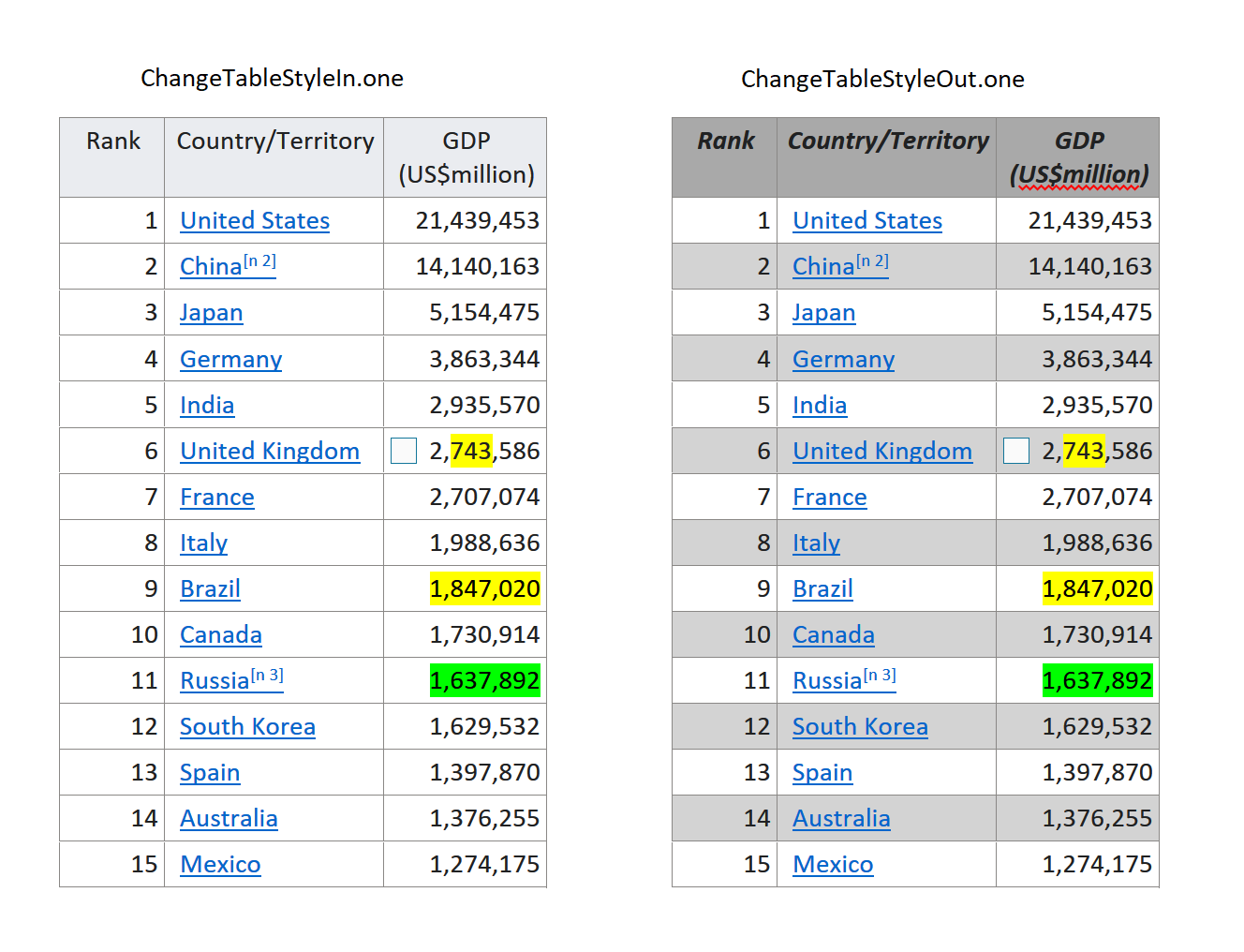