Creating Car Body Drawing
Creating Car Body Drawing
The Aspose.Drawing .NET (C#) 2d graphic library provides designers with a powerful tool for creating captivating drawings. You can combine different images and text strings with semi-transparency to create unique drawings. In the following example, we'll take a car image, coloring the body and incorporate a logo image with text, blending them on the car's body. By using semi-transparency in the blending process, we can maintain the visibility of reflections on the car's body surface. We'll apply color to the car by utilizing a semi-transparent layer and a clipping region that covers only the car body, excluding the wheels and windows. Moreover, we'll simulate the process of applying and blending various "skins" with a laser beam to showcase a dynamic transition between these skins.
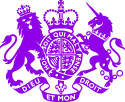
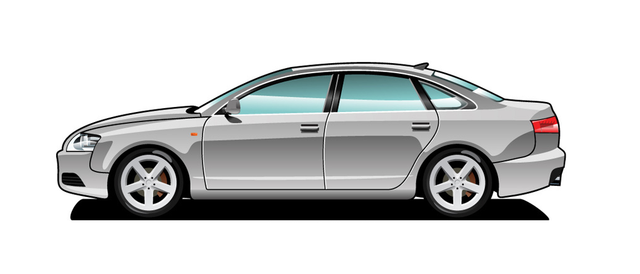
To demonstrate the drawing capabilities, let's create various skin designs for the car's body image. The `Skin` class comprises a background color, and within it, the `DesignElement` class further consists of a `Banner` class containing a text string and a `Logo` class with image attributes. Now you can assign different skins with background colors using the Color.FromArgb method. The `FromArgb()` method creates a new skin color with parameters and the first one is the alpha layer to determine transparency levels. So, skins number 1 and 2 in the code example below will use only a colored background, while skins 3 and 4 use additional `DesignElements` with a logo image, a position, and the banner text strings "KEWIN" and "ANCHOR," respectively. Define a font name, size, style and color per each `Banner` element:
Car body coloration
Next, we create a `GraphicsPath` object named `carBody`, which outlines the car image using Bezier curves. We then incorporate a new Bitmap frame created from both the `carBody` and the skin using the `DrawFrame` function:
The `DrawFrame` function creates new `Bitmap` graphics with dimensions `w x h` and a white background color. We enable graphics smoothing with high quality and text rendering hinting with anti-aliasing attributes. Following that, we load a source car body PNG image file and use the Graphics.DrawImage method to render it onto the bitmap. Additionally, we fill the interior of the car body's `GraphicsPath` with the skin's background color using the FillPath method. This way, the background color will only be applied to the region defined by the `carBody` outline:
If our skin includes a `Banner` text and a `Logo` image, we will also render them onto the graphics using the DrawString and DrawImage methods. We use Graphics.Clip property to ensure that the drawn text string and image logo are confined to the clip region corresponding to the `carBody` outline:
Transparent bitmap blending
Now, we have two bitmaps: one featuring a colored car body and the second representing a text string and logo image. To blend these bitmaps while preserving semi-transparency, we can employ the `DrawImage` method, but with an added ImageAttributes parameter. We create a ColorMatrix where one of its elements, responsible for transparency, is set to a value of 0.7. This matrix is then utilized as an image attribute using the SetColorMatrix method:
Mixing two bitmaps
Furthermore, we can mix bitmap frames that feature various skins into a sequence of images, which can then be saved to files in the PNG format. This allows two skins to be simultaneously blended into the car body image, separated by an edge line. To create a mixed bitmap we assign two objects `frame1` and `frame2` with current and next bitmaps skins:
We will mix two bitmaps with different skins to create a new one, gradually changing the proportion from 0% to 100%. On this resulting bitmap, we will use the DrawImage method to draw the left portion from 'frame1' and the right portion from 'frame2', divided by a vertical edge line whose position is determined by the 'percent' variable:
Drawing laser edge line
Additionally, we will draw a laser vertical line between the two bitmaps, which will be 5 pixels wide. To achieve this, we create a rectangle at a position that corresponds to the percentage of the skin bitmaps mixing proportion. We then obtain the region, which is an intersection of our rectangle and the `carBody` graphics path, using the Region.Intersect method. Finally, we fill this region with a magenta-colored brush.
Resulting drawings
By utilizing a straightforward Aspose.Drawing API to draw images and text strings, fill regions with semi-transparent colors, and blend bitmaps, we've crafted complex drawings. Thanks to the transparency, the resulting car body images take on new colors, as do the logo image and text drawings. In the example drawing below, you can observe two skins simultaneously applied to the car body, separated by a simulated laser beam:
Showcase video
Finally, you can convert the series of PNG images with mixed skins into MP4 video with 30 frames per second and H.264 codec using `ffmpeg` program:
ffmpeg -framerate 30 -i ./out/%%05d.png -vcodec libx264 -pix_fmt yuv420p ./out/CarBody.mp4
Source code
Please see the full C# code example on the Aspose.Drawing Github: CarBody.cs