ドキュメントのプロパティを操作する
ドキュメントのプロパティを使用すると、ドキュメントに関するいくつかの有用な情報を保存できます。これらのプロパティは、次の 2 つのグループに分類できます。
- ドキュメントのタイトル、作成者名、ドキュメントの統計などの値を含むシステムまたは組み込み。
- ユーザー定義またはカスタム。ユーザーが名前と値の両方を定義できる名前と値のペアとして提供されます。
API とバージョン番号に関する情報が出力ドキュメントに直接書き込まれることを知っておくと便利です。たとえば、ドキュメントを PDF に変換すると、Aspose.Words は「アプリケーション」フィールドに「Aspose.Words」を入力し、「PDF プロデューサー」フィールドに「Aspose.Words for .NET YY.MN」を入力します。ここで、YY.M.N は変換に使用された Aspose.Words のバージョンです。 。詳細については、「出力ドキュメントに含まれるジェネレーターまたはプロデューサーの名前」を参照してください。
ドキュメントのプロパティにアクセスする
Aspose.Words でドキュメントのプロパティにアクセスするには、次を使用します。
-
BuiltInDocumentProperties は組み込みプロパティを取得します。
-
カスタム プロパティを取得するための CustomDocumentProperties。
BuiltInDocumentProperties と CustomDocumentProperties は DocumentProperty オブジェクトのコレクションです。これらのオブジェクトは、インデクサー プロパティを通じて名前またはインデックスによって取得できます。
さらに、BuiltInDocumentProperties は、適切なタイプの値を返す入力されたプロパティのセットを通じてドキュメント プロパティへのアクセスを提供します。 CustomDocumentProperties を使用すると、ドキュメントのプロパティをドキュメントに追加または削除できます。
DocumentProperty クラスを使用すると、ドキュメント プロパティの名前、値、およびタイプを取得できます。 Value はオブジェクトを返しますが、特定の型に変換されたプロパティ値を取得できる一連のメソッドがあります。プロパティの型がわかったら、DocumentProperty.ToString や DocumentProperty.ToInt などの DocumentProperty.ToXXX メソッドの 1 つを使用して、適切な型の値を取得できます。
次のコード例は、ドキュメント内のすべての組み込みプロパティとカスタム プロパティを列挙する方法を示しています。
// For complete examples and data files, please go to https://github.com/aspose-words/Aspose.Words-for-.NET | |
string fileName = dataDir + "Properties.doc"; | |
Document doc = new Document(fileName); | |
Console.WriteLine("1. Document name: {0}", fileName); | |
Console.WriteLine("2. Built-in Properties"); | |
foreach (DocumentProperty prop in doc.BuiltInDocumentProperties) | |
Console.WriteLine("{0} : {1}", prop.Name, prop.Value); | |
Console.WriteLine("3. Custom Properties"); | |
foreach (DocumentProperty prop in doc.CustomDocumentProperties) | |
Console.WriteLine("{0} : {1}", prop.Name, prop.Value); |
Microsoft Word では、「ファイル → プロパティ」メニューを使用してドキュメントのプロパティにアクセスできます。
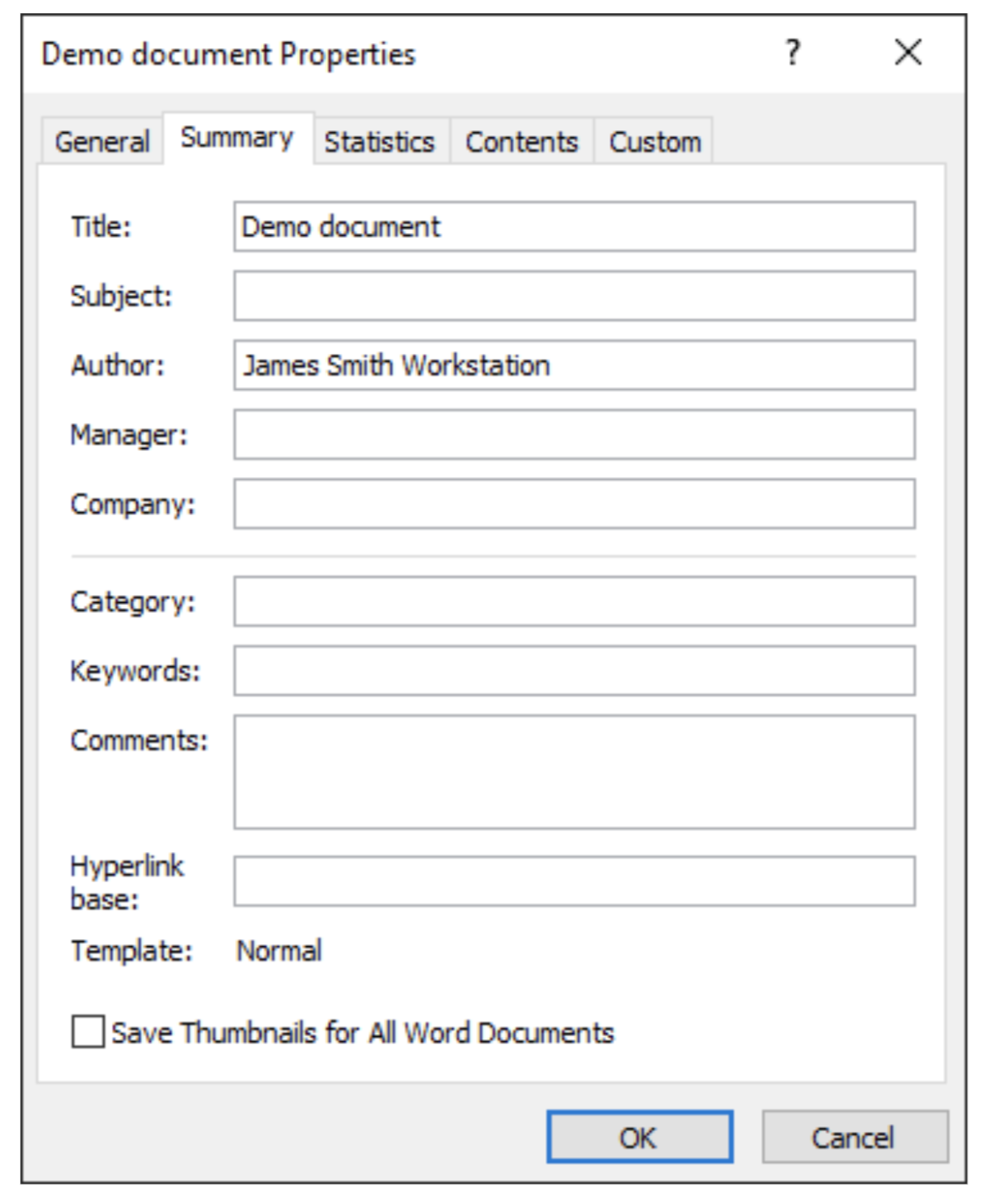
ドキュメントのプロパティの追加または削除
Aspose.Words を使用して組み込みドキュメント プロパティを追加または削除することはできません。値の変更または更新のみが可能です。
Aspose.Words でカスタム ドキュメント プロパティを追加するには、Add メソッドを使用して、新しいプロパティ名と適切な型の値を渡します。このメソッドは、新しく作成された DocumentProperty オブジェクトを返します。
カスタム プロパティを削除するには、Remove メソッドを使用して削除するプロパティ名を渡すか、RemoveAt メソッドを使用してインデックスによってプロパティを削除します。 Clear メソッドを使用してすべてのプロパティを削除することもできます。
次のコード例では、指定された名前のカスタム プロパティがドキュメント内に存在するかどうかを確認し、さらにいくつかのカスタム ドキュメント プロパティを追加します。
// For complete examples and data files, please go to https://github.com/aspose-words/Aspose.Words-for-.NET | |
Document doc = new Document(dataDir + "Properties.doc"); | |
CustomDocumentProperties props = doc.CustomDocumentProperties; | |
if (props["Authorized"] == null) | |
{ | |
props.Add("Authorized", true); | |
props.Add("Authorized By", "John Smith"); | |
props.Add("Authorized Date", DateTime.Today); | |
props.Add("Authorized Revision", doc.BuiltInDocumentProperties.RevisionNumber); | |
props.Add("Authorized Amount", 123.45); | |
} |
次のコード例は、カスタム ドキュメント プロパティを削除する方法を示しています。
// For complete examples and data files, please go to https://github.com/aspose-words/Aspose.Words-for-.NET | |
Document doc = new Document(dataDir + "Properties.doc"); | |
doc.CustomDocumentProperties.Remove("Authorized Date"); |
組み込みのドキュメントプロパティを更新する
Aspose.Words は、Microsoft Word の一部のプロパティとは異なり、ドキュメント プロパティを自動的に更新しませんが、一部の統計的な組み込みドキュメント プロパティを更新する方法を提供します。 UpdateWordCount メソッドを呼び出して、次のプロパティを再計算して更新します。
コンテンツにリンクされた新しいカスタム プロパティを作成する
Aspose.Words は、コンテンツにリンクされた新しいカスタム ドキュメント プロパティを作成する AddLinkToContent メソッドを提供します。このプロパティは、新しく作成されたプロパティ オブジェクトを返すか、LinkSource が無効な場合は null を返します。
次のコード例は、カスタム プロパティへのリンクを構成する方法を示しています。
// For complete examples and data files, please go to https://github.com/aspose-words/Aspose.Words-for-.NET | |
Document doc = new Document(dataDir + "test.docx"); | |
// Retrieve a list of all custom document properties from the file. | |
CustomDocumentProperties customProperties = doc.CustomDocumentProperties; | |
// Add linked to content property. | |
DocumentProperty customProperty = customProperties.AddLinkToContent("PropertyName", "BookmarkName"); | |
// Also, accessing the custom document property can be performed by using the property name. | |
customProperty = customProperties["PropertyName"]; | |
// Check whether the property is linked to content. | |
bool isLinkedToContent = customProperty.IsLinkToContent; | |
// Get the source of the property. | |
string source = customProperty.LinkSource; | |
// Get the value of the property. | |
string value = customProperty.Value.ToString(); |
ドキュメント変数の取得
Variables プロパティを使用してドキュメント変数のコレクションを取得できます。変数名と値は文字列です。
次のコード例は、ドキュメント変数を列挙する方法を示しています。
// For complete examples and data files, please go to https://github.com/aspose-words/Aspose.Words-for-.NET | |
// The path to the documents directory. | |
string dataDir = RunExamples.GetDataDir_WorkingWithDocument(); | |
// Load the template document. | |
Document doc = new Document(dataDir + "TestFile.doc"); | |
string variables = ""; | |
foreach (KeyValuePair<string, string> entry in doc.Variables) | |
{ | |
string name = entry.Key.ToString(); | |
string value = entry.Value.ToString(); | |
if (variables == "") | |
{ | |
// Do something useful. | |
variables = "Name: " + name + "," + "Value: {1}" + value; | |
} | |
else | |
{ | |
variables = variables + "Name: " + name + "," + "Value: {1}" + value; | |
} | |
} |
文書から個人情報を削除する
Word 文書を他の人と共有する場合は、作成者名や会社などの個人情報を削除したい場合があります。これを行うには、RemovePersonalInformation プロパティを使用して、文書の保存時に Microsoft Word がコメント、リビジョン、および文書プロパティからすべてのユーザー情報を削除することを示すフラグを設定します。
次のコード例は、個人情報を削除する方法を示しています。
// For complete examples and data files, please go to https://github.com/aspose-words/Aspose.Words-for-.NET | |
Document doc = new Document(dataDir + "Properties.doc"); | |
doc.RemovePersonalInformation = true; | |
dataDir = dataDir + "RemovePersonalInformation_out.docx"; | |
doc.Save(dataDir); |