Working with Vector Graphics in Java
Contents
[
Hide
]
Creating vector graphics in Java becomes a straightforward task with Aspose.Drawing library for Java. This API facilitates working with a diverse range of vector graphics, including arcs, Bezier splines, Cardinal splines, closed curves, ellipses, lines, and various other types. The following Java examples demonstrate how to draw different types of vector graphics using this API.
Draw Arc in Java
To draw an arc using Java:
- Create an object of
Bitmap
class. - Initialize an object of
Graphics
class from this bitmap - Define a
Pen
object with desired parameters - Use the
drawArc()
method ofGraphics
class object to draw an arc
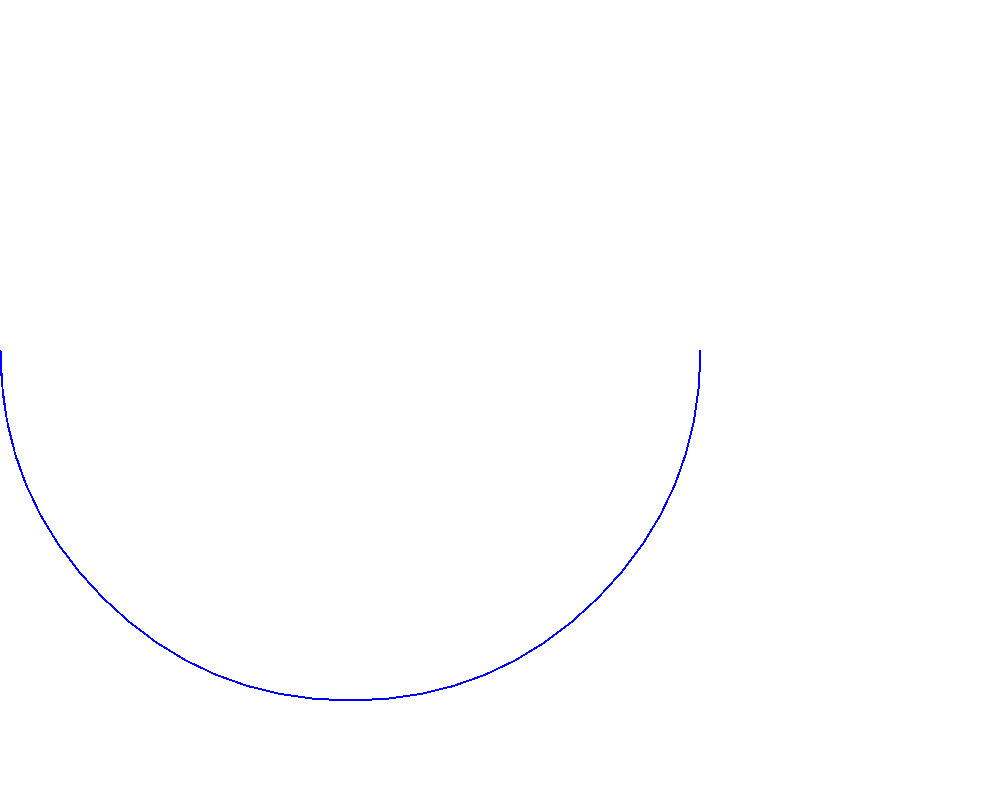
Draw Bezier Spline in Java
- Create an object of
Bitmap
class. - Initialize an object of
Graphics
class from this bitmap - Define a
Pen
object with desired parameters - Use the
drawBezier()
method ofGraphics
class object to draw Bezier Spline
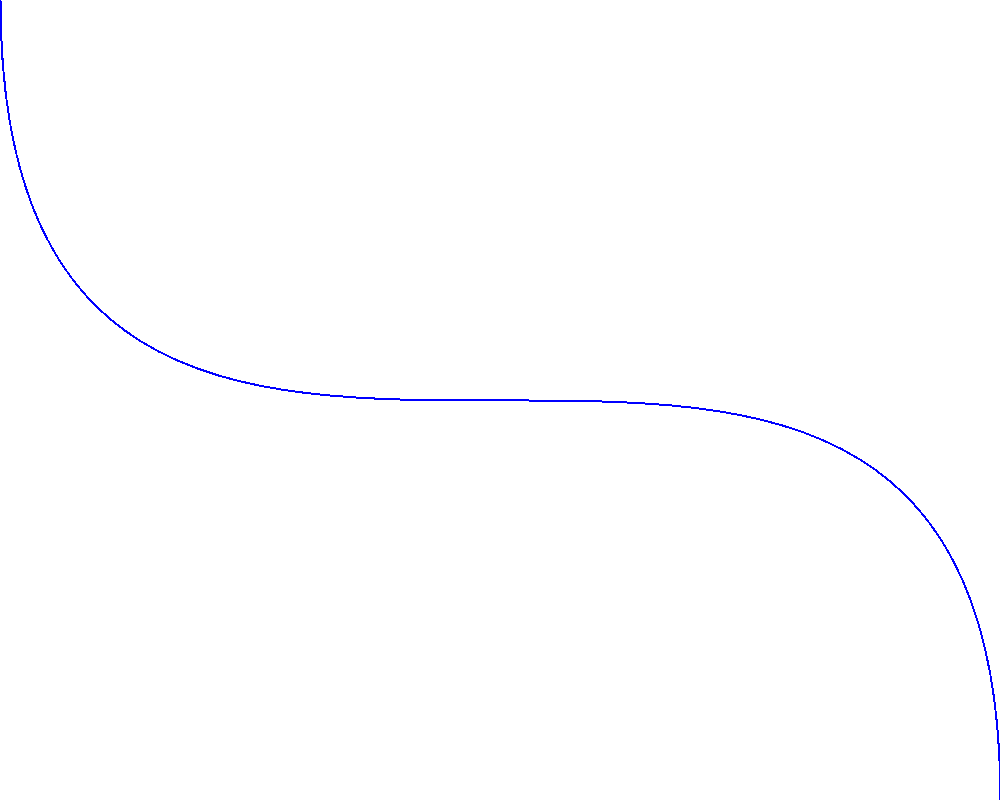
Draw Cardinal Spline in Java
- Create an object of
Bitmap
class. - Initialize an object of
Graphics
class from this bitmap - Define a
Pen
object with desired parameters - Use the
drawCurve()
method ofGraphics
class object to draw Cardinal Spline
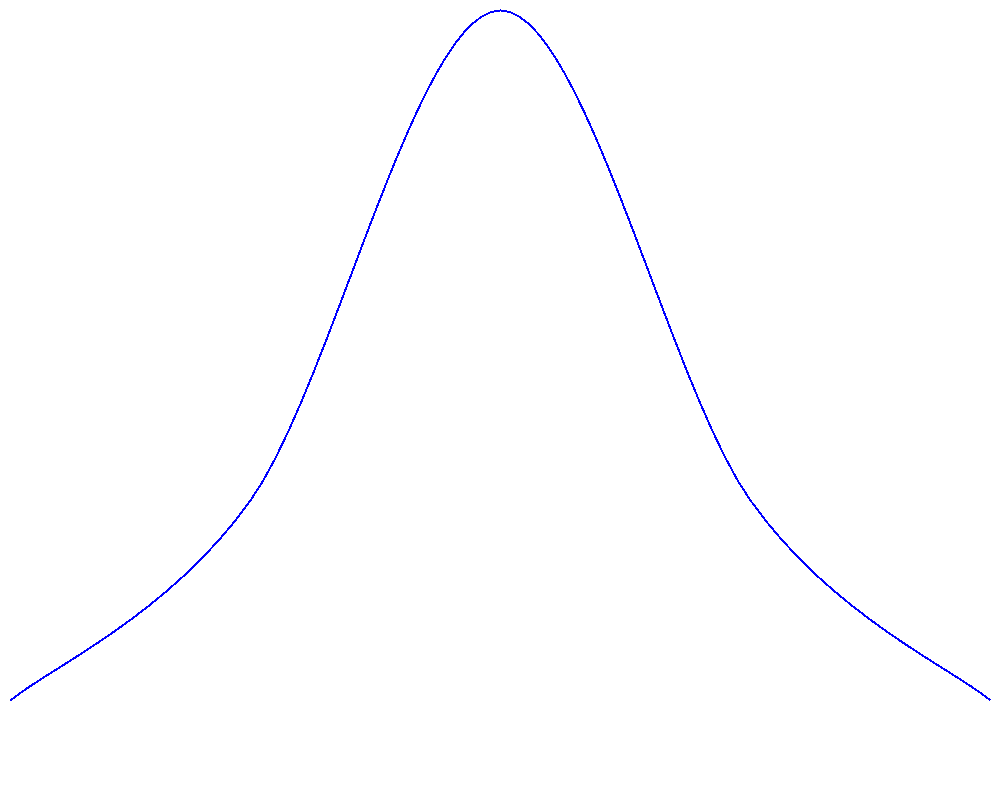
Draw Closed Curve in Java
- Create an object of
Bitmap
class. - Initialize an object of
Graphics
class from this bitmap - Define a
Pen
object with desired parameters - Use the
drawClosedCurve()
method ofGraphics
class object to draw a closed curve
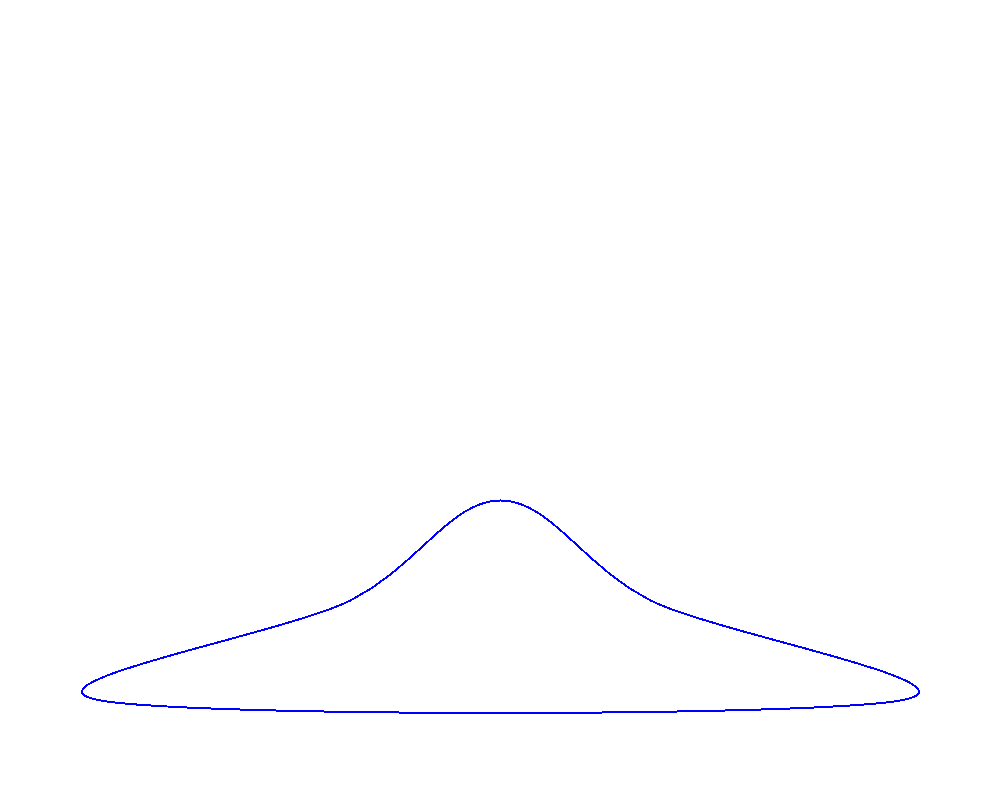
Draw Ellipse in Java
- Create an object of
Bitmap
class. - Initialize an object of
Graphics
class from this bitmap. - Define a
Pen
object with desired parameters - Use the
drawEllipse()
method ofGraphics
class object to draw Ellipse
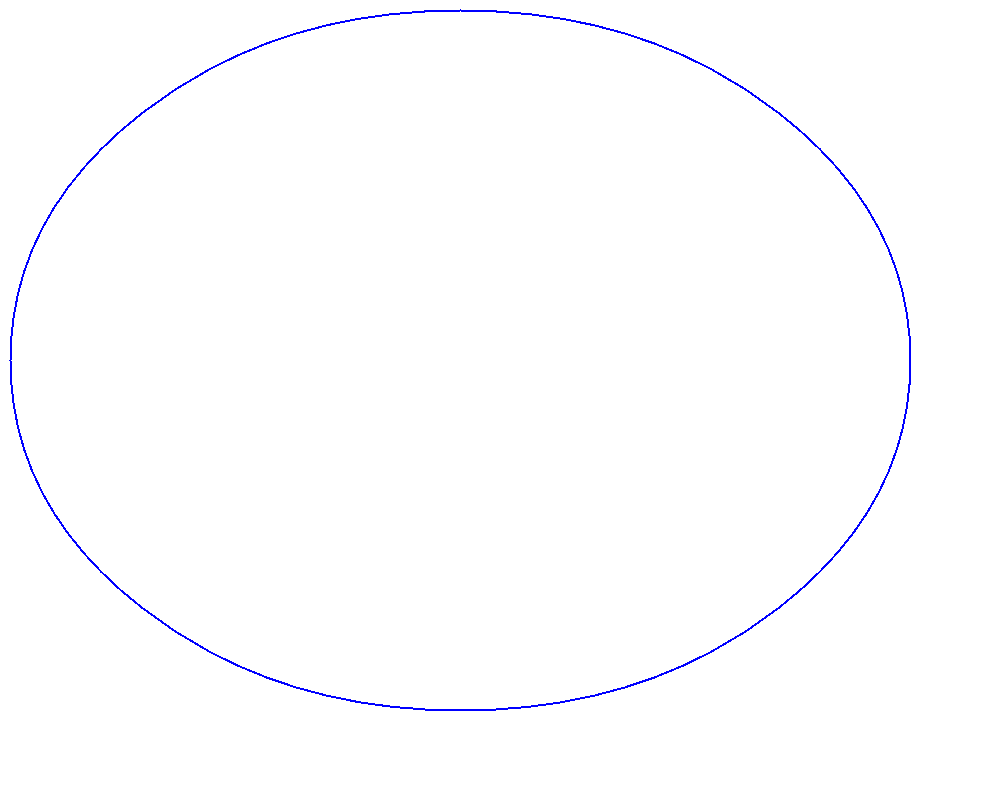
Draw Lines in Java
- Create an object of
Bitmap
class. - Initialize an object of
Graphics
class from this bitmap - Define a
Pen
object with desired parameters - Use the
drawLine()
method ofGraphics
class object to draw lines
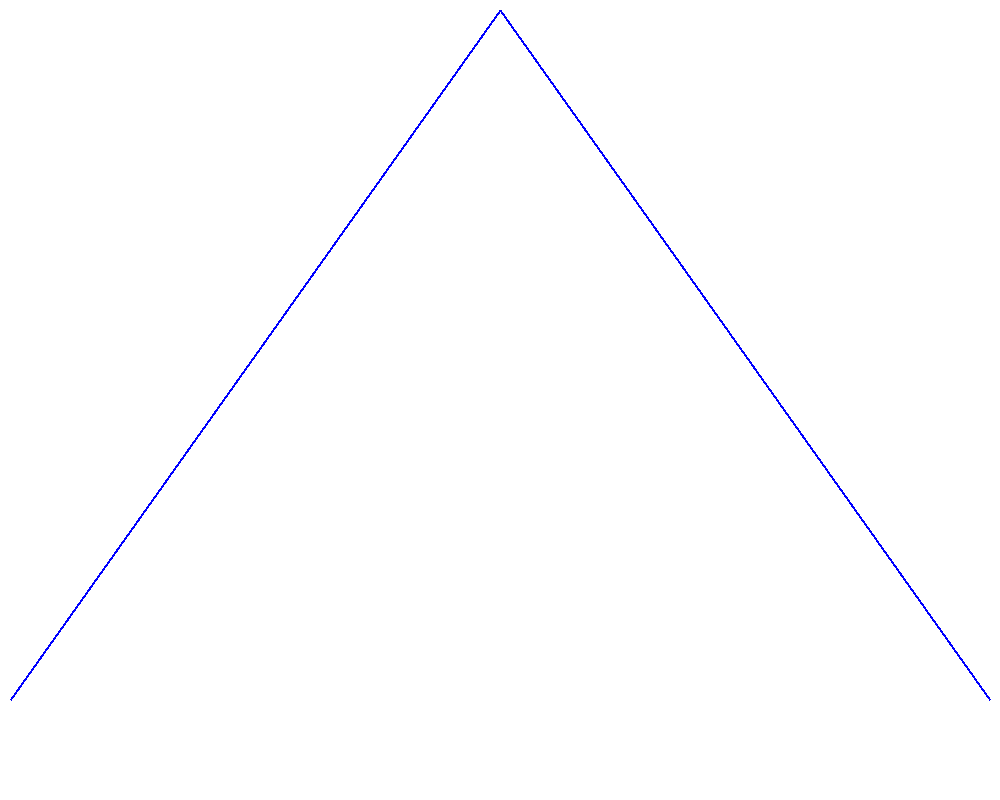
Draw Path in Java
- Create an object of
Bitmap
class. - Initialize an object of
Graphics
class from this bitmap - Define a
Pen
object with desired parameters - Initialize a new object of
GraphicsPath
class and add Lines to its path collection
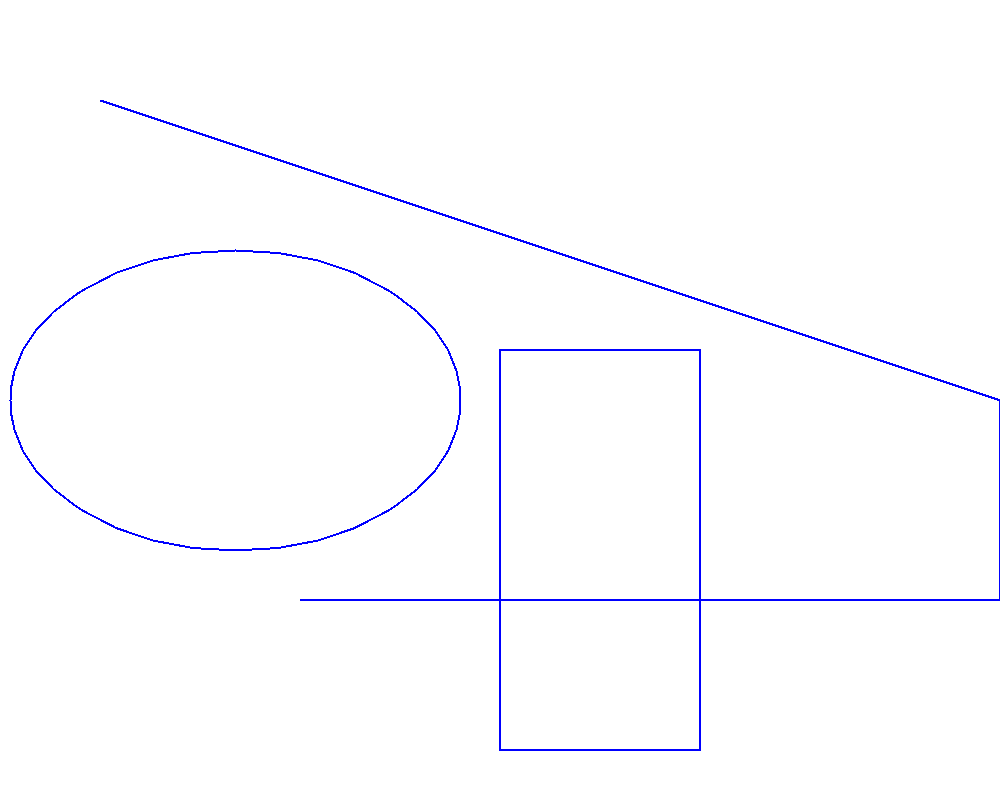
Draw Polygon in Java
- Create an object of
Bitmap
class. - Initialize an object of
Graphics
class from this bitmap - Define a
Pen
object with desired parameters - Use the
drawPolygon()
method ofGraphics
class object to draw Polygon
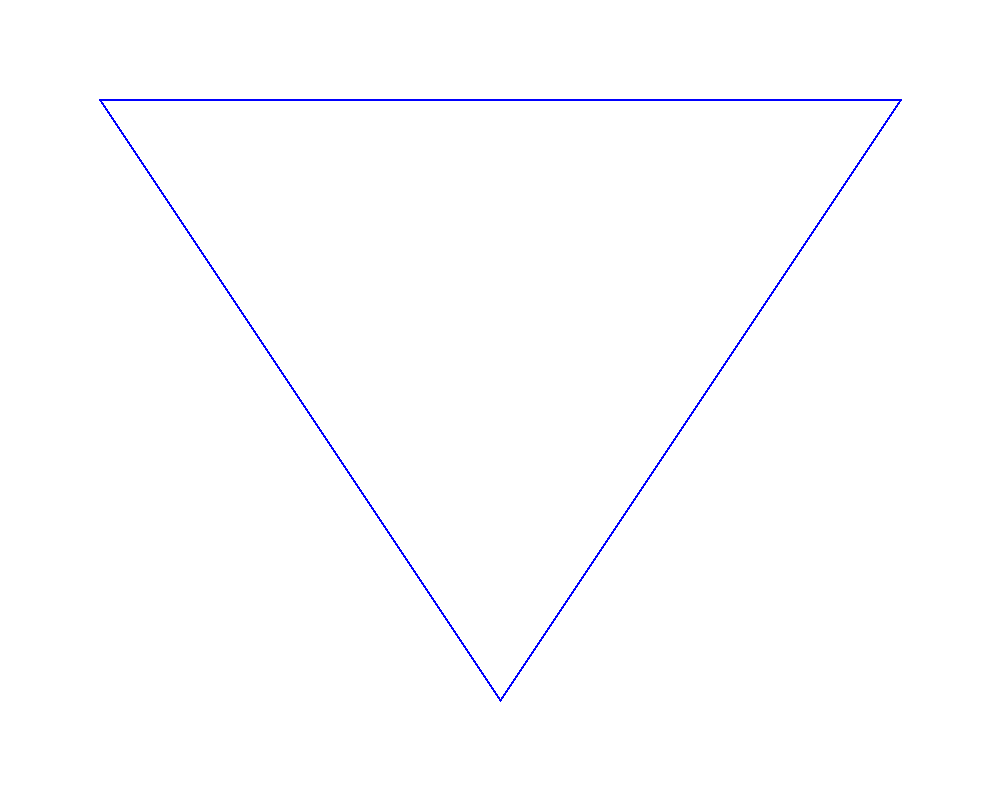
Draw Rectangle in Java
- Create an object of
Bitmap
class. - Initialize an object of
Graphics
class from this bitmap - Define a
Pen
object with desired parameters - Use the
drawRectangle()
method ofGraphics
class object to draw Rectangle
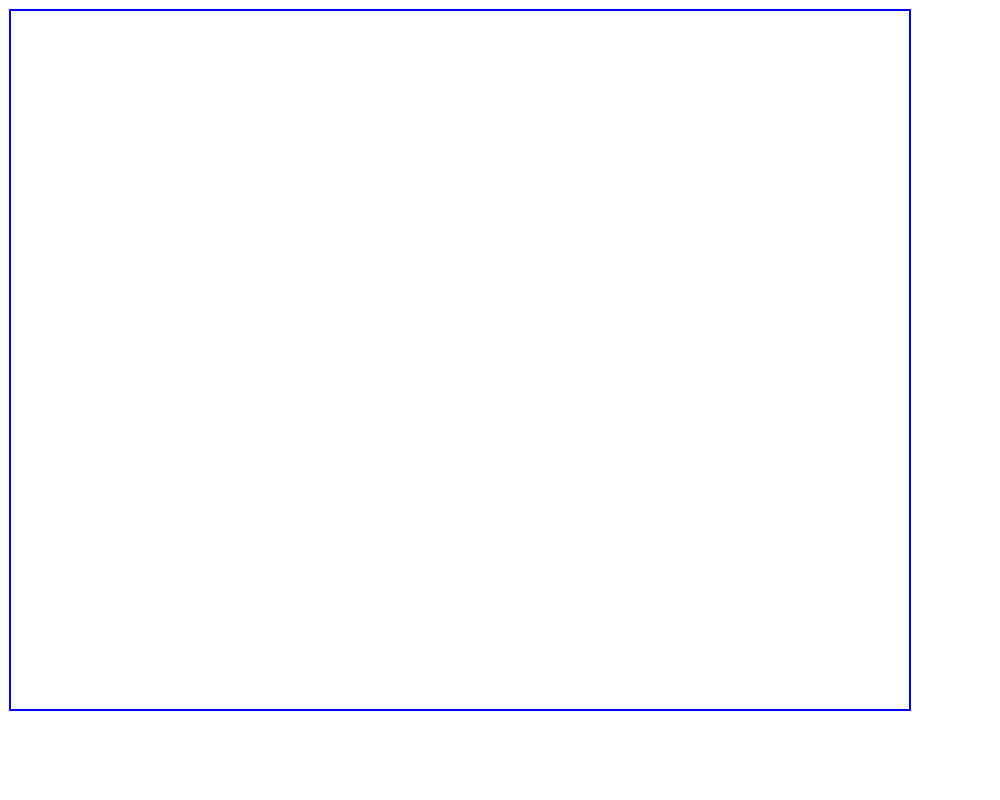
Fill Region in Java
- Create an object of
Bitmap
class. - Initialize an object of
Graphics
class from this bitmap - Create a
GraphicsPath
class class object - Add polygon to the Path collection of
GraphicsPath
class object - Use the
fillRegion()
method ofGraphics
class to fill defined regions
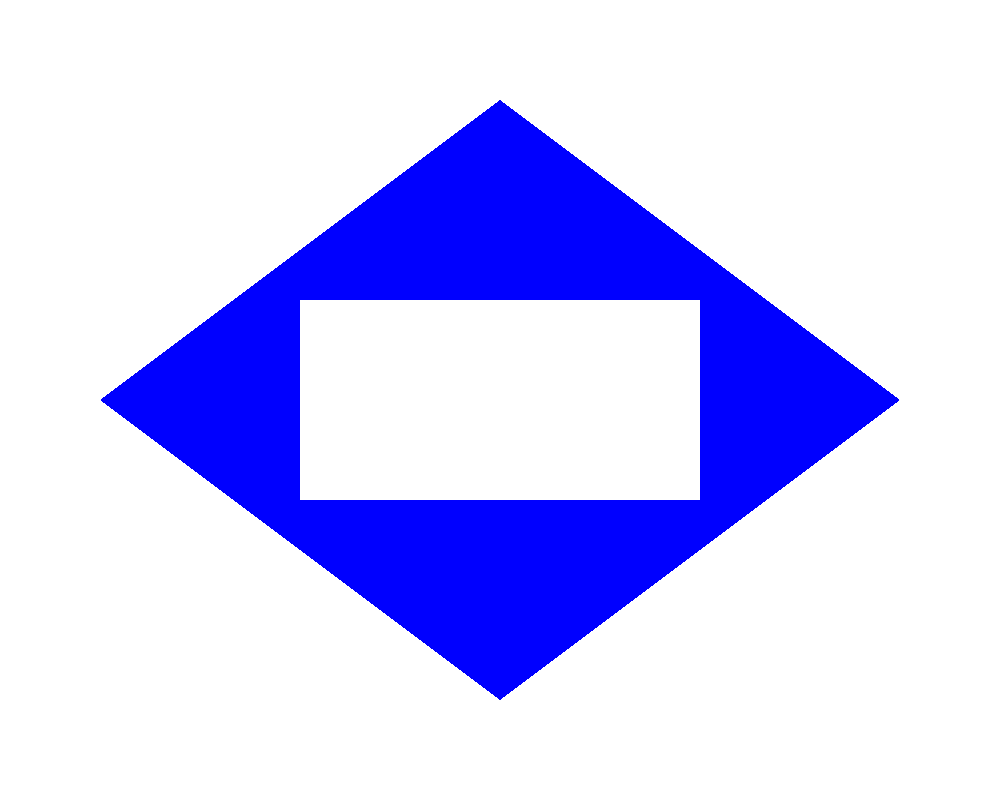