列と行の操作
テーブルの動作をより詳細に制御するには、列と行を操作する方法を学習します。
テーブル要素インデックスの検索
列、行、およびセルは、選択したドキュメントノードにインデックスでアクセスすることによって管理されます。 ノードのインデックスを見つけるには、親ノードから要素タイプのすべての子ノードを収集し、IndexOfメソッドを使用してコレクション内の目的のノードのイン
ドキュメント内のテーブルのインデックスを検索する
場合によっては、ドキュメント内の特定のテーブルに変更を加える必要があることがあります。 これを行うには、そのインデックスでテーブルを参照できます。
次のコード例は、ドキュメント内のテーブルのインデックスを取得する方法を示しています:
// For complete examples and data files, please go to https://github.com/aspose-words/Aspose.Words-for-Java.git. | |
Table table = (Table) doc.getChild(NodeType.TABLE, 0, true); | |
NodeCollection allTables = doc.getChildNodes(NodeType.TABLE, true); | |
int tableIndex = allTables.indexOf(table); |
テーブル内の行のインデックスの検索
同様に、選択したテーブルの特定の行に変更を加える必要がある場合があります。 これを行うには、そのインデックスで行を参照することもできます。
次のコード例は、テーブル内の行のインデックスを取得する方法を示しています:
// For complete examples and data files, please go to https://github.com/aspose-words/Aspose.Words-for-Java.git. | |
int rowIndex = table.indexOf(table.getLastRow()); |
行内のセルのインデックスの検索
最後に、特定のセルに変更を加える必要がある場合があり、セルインデックスでもこれを行うことができます。
次のコード例は、行のセルのインデックスを取得する方法を示しています:
// For complete examples and data files, please go to https://github.com/aspose-words/Aspose.Words-for-Java.git. | |
int cellIndex = row.indexOf(row.getCells().get(4)); |
列の操作
Aspose.Wordsドキュメントオブジェクトモデル(DOM)では、TableノードはRowノードとCellノードで構成されます。 したがって、Aspose.WordsのDocument
オブジェクトモデルでは、Word文書のように、列の概念はありません。
設計上、Microsoft WordとAspose.Wordsのテーブル行は完全に独立しており、基本的なプロパティと操作はテーブルの行とセルにのみ含まれています。 これにより、テーブルにいくつかの興味深い属性を持たせることができます:
- 各テーブルの行には、完全に異なる数のセルを含めることができます
- 垂直方向には、各行のセルの幅が異なる場合があります
- 異なる行形式とセル数のテーブルを結合することができます
列に対して実行される操作は、実際には、列に適用されているように見えるように行セルをまとめて変更することによって操作を実行する「ショートカット」です。 つまり、同じテーブル行のセルインデックスを反復処理するだけで、列に対して操作を実行できます。
次のコード例では、テーブルの"列"を構成するセルを収集するファサードクラスを証明することにより、このような操作を簡素化します:
// For complete examples and data files, please go to https://github.com/aspose-words/Aspose.Words-for-Java.git. | |
/// <summary> | |
/// Represents a facade object for a column of a table in a Microsoft Word document. | |
/// </summary> | |
static class Column | |
{ | |
private Column(Table table, int columnIndex) { | |
if (table != null) { | |
mTable = table; | |
} else { | |
throw new IllegalArgumentException("table"); | |
} | |
mColumnIndex = columnIndex; | |
} | |
/// <summary> | |
/// Returns a new column facade from the table and supplied zero-based index. | |
/// </summary> | |
public static Column fromIndex(Table table, int columnIndex) | |
{ | |
return new Column(table, columnIndex); | |
} | |
private ArrayList<Cell> getCells() { | |
return getColumnCells(); | |
} | |
/// <summary> | |
/// Returns the index of the given cell in the column. | |
/// </summary> | |
public int indexOf(Cell cell) | |
{ | |
return getColumnCells().indexOf(cell); | |
} | |
/// <summary> | |
/// Inserts a brand new column before this column into the table. | |
/// </summary> | |
public Column insertColumnBefore() | |
{ | |
ArrayList<Cell> columnCells = getCells(); | |
if (columnCells.size() == 0) | |
throw new IllegalArgumentException("Column must not be empty"); | |
// Create a clone of this column. | |
for (Cell cell : columnCells) | |
cell.getParentRow().insertBefore(cell.deepClone(false), cell); | |
// This is the new column. | |
Column column = new Column(columnCells.get(0).getParentRow().getParentTable(), mColumnIndex); | |
// We want to make sure that the cells are all valid to work with (have at least one paragraph). | |
for (Cell cell : column.getCells()) | |
cell.ensureMinimum(); | |
// Increase the index which this column represents since there is now one extra column in front. | |
mColumnIndex++; | |
return column; | |
} | |
/// <summary> | |
/// Removes the column from the table. | |
/// </summary> | |
public void remove() | |
{ | |
for (Cell cell : getCells()) | |
cell.remove(); | |
} | |
/// <summary> | |
/// Returns the text of the column. | |
/// </summary> | |
public String toTxt() throws Exception | |
{ | |
StringBuilder builder = new StringBuilder(); | |
for (Cell cell : getCells()) | |
builder.append(cell.toString(SaveFormat.TEXT)); | |
return builder.toString(); | |
} | |
/// <summary> | |
/// Provides an up-to-date collection of cells which make up the column represented by this facade. | |
/// </summary> | |
private ArrayList<Cell> getColumnCells() | |
{ | |
ArrayList<Cell> columnCells = new ArrayList<Cell>(); | |
for (Row row : mTable.getRows()) | |
{ | |
Cell cell = row.getCells().get(mColumnIndex); | |
if (cell != null) | |
columnCells.add(cell); | |
} | |
return columnCells; | |
} | |
private int mColumnIndex; | |
private Table mTable; | |
} |
次のコード例は、テーブルに空白の列を挿入する方法を示しています:
// For complete examples and data files, please go to https://github.com/aspose-words/Aspose.Words-for-Java.git. | |
Document doc = new Document(getMyDir() + "Tables.docx"); | |
Table table = (Table) doc.getChild(NodeType.TABLE, 0, true); | |
Column column = Column.fromIndex(table, 0); | |
// Print the plain text of the column to the screen. | |
System.out.println(column.toTxt()); | |
// Create a new column to the left of this column. | |
// This is the same as using the "Insert Column Before" command in Microsoft Word. | |
Column newColumn = column.insertColumnBefore(); | |
for (Cell cell : newColumn.getColumnCells()) | |
cell.getFirstParagraph().appendChild(new Run(doc, "Column Text " + newColumn.indexOf(cell))); |
次のコード例は、ドキュメント内のテーブルから列を削除する方法を示しています:
// For complete examples and data files, please go to https://github.com/aspose-words/Aspose.Words-for-Java.git. | |
Document doc = new Document(getMyDir() + "Tables.docx"); | |
Table table = (Table) doc.getChild(NodeType.TABLE, 1, true); | |
Column column = Column.fromIndex(table, 2); | |
column.remove(); |
行をヘッダー行として指定する
テーブルの最初の行をヘッダー行として最初のページでのみ繰り返すか、テーブルが複数に分割されている場合は各ページで繰り返すかを選択できます。 Aspose.Wordsでは、HeadingFormatプロパティを使用してすべてのページでヘッダー行を繰り返すことができます。
そのような行がテーブルの先頭に次々に配置されている場合は、複数のヘッダー行にマークを付けることもできます。 これを行うには、これらの行にHeadingFormatプロパティを適用する必要があります。
次のコード例は、後続のページで繰り返されるヘッダー行を含むテーブルを作成する方法を示しています:
// For complete examples and data files, please go to https://github.com/aspose-words/Aspose.Words-for-Java.git. | |
Document doc = new Document(); | |
DocumentBuilder builder = new DocumentBuilder(doc); | |
builder.startTable(); | |
builder.getRowFormat().setHeadingFormat(true); | |
builder.getParagraphFormat().setAlignment(ParagraphAlignment.CENTER); | |
builder.getCellFormat().setWidth(100.0); | |
builder.insertCell(); | |
builder.writeln("Heading row 1"); | |
builder.endRow(); | |
builder.insertCell(); | |
builder.writeln("Heading row 2"); | |
builder.endRow(); | |
builder.getCellFormat().setWidth(50.0); | |
builder.getParagraphFormat().clearFormatting(); | |
for (int i = 0; i < 50; i++) | |
{ | |
builder.insertCell(); | |
builder.getRowFormat().setHeadingFormat(false); | |
builder.write("Column 1 Text"); | |
builder.insertCell(); | |
builder.write("Column 2 Text"); | |
builder.endRow(); | |
} | |
doc.save(getArtifactsDir() + "WorkingWithTables.RepeatRowsOnSubsequentPages.docx"); |
テーブルと行がページ間で分割されないようにする
テーブルの内容をページ間で分割してはならない場合があります。 たとえば、タイトルがテーブルの上にある場合、適切な外観を維持するために、タイトルとテーブルは常に同じページにまとめておく必要があります。
この機能を実現するのに便利な2つの別々の手法があります:
Allow row break across pages
は、テーブル行に適用されますKeep with next
は、表のセルの段落に適用されます
デフォルトでは、上記のプロパティは無効になっています。
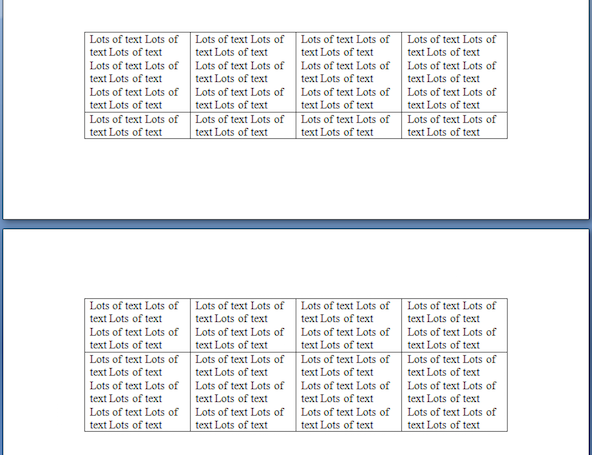
行がページ {#keep-a-row-from-breaking-across-pages}を越えて破損しないようにする
これには、行のセル内のコンテンツがページ間で分割されないように制限することが含まれます。 Microsoft Wordでは、これはテーブルのプロパティの下に"行をページ間で分割することを許可する"オプションとしてあります。 Aspose.Wordsでは、これはRowのRowFormatオブジェクトの下にプロパティRowFormat.AllowBreakAcrossPagesとしてあります。
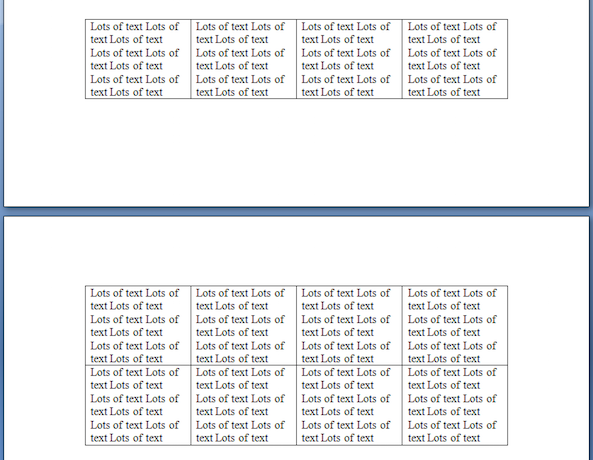
次のコード例は、テーブル内の各行のページ間で行を分割することを無効にする方法を示しています:
// For complete examples and data files, please go to https://github.com/aspose-words/Aspose.Words-for-Java.git. | |
Document doc = new Document(getMyDir() + "Table spanning two pages.docx"); | |
Table table = (Table) doc.getChild(NodeType.TABLE, 0, true); | |
// Disable breaking across pages for all rows in the table. | |
for (Row row : (Iterable<Row>) table.getRows()) | |
row.getRowFormat().setAllowBreakAcrossPages(false); | |
doc.save(getArtifactsDir() + "WorkingWithTables.RowFormatDisableBreakAcrossPages.docx"); |
テーブルがページ間で破壊されないようにする
テーブルがページ間で分割されないようにするには、テーブル内に含まれるコンテンツを一緒に保つように指定する必要があります。
これを行うために、Aspose.Wordsは、ユーザーがテーブルを選択し、テーブルセル内の各段落に対してKeepWithNextパラメータをtrueに有効にするメソッドを使用します。 例外は、テーブルの最後の段落であり、falseに設定する必要があります。
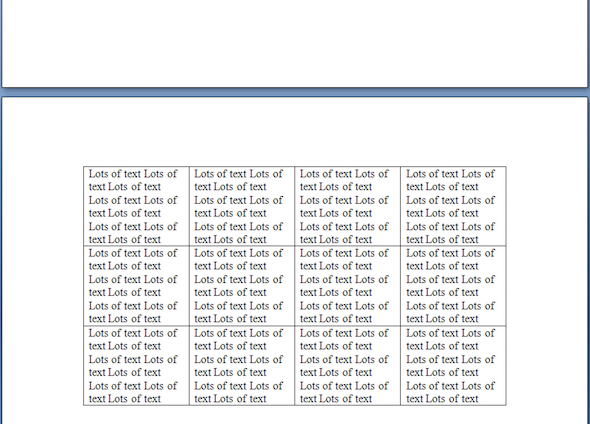
次のコード例は、同じページに一緒に滞在するようにテーブルを設定する方法を示しています:
// For complete examples and data files, please go to https://github.com/aspose-words/Aspose.Words-for-Java.git. | |
Document doc = new Document(getMyDir() + "Table spanning two pages.docx"); | |
Table table = (Table) doc.getChild(NodeType.TABLE, 0, true); | |
// We need to enable KeepWithNext for every paragraph in the table to keep it from breaking across a page, | |
// except for the last paragraphs in the last row of the table. | |
for (Cell cell : (Iterable<Cell>) table.getChildNodes(NodeType.CELL, true)) | |
{ | |
cell.ensureMinimum(); | |
for (Paragraph para : (Iterable<Paragraph>) cell.getParagraphs()) | |
if (!(cell.getParentRow().isLastRow() && para.isEndOfCell())) | |
para.getParagraphFormat().setKeepWithNext(true); | |
} | |
doc.save(getArtifactsDir() + "WorkingWithTables.KeepTableTogether.docx"); |